Fallback image if image is not found in picture source tag
How to do graceful degradation if source image not found in picture tag
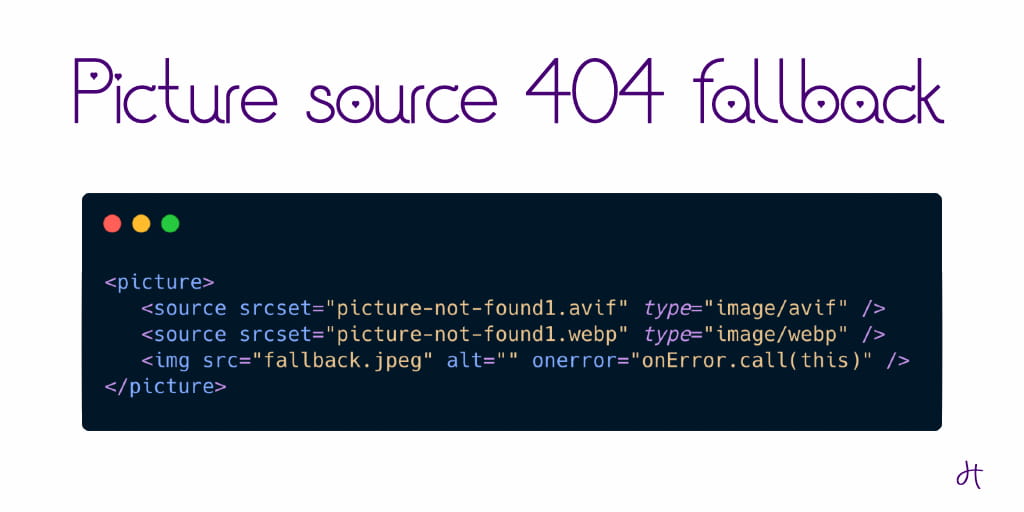
When dealing with a large collection of image paths from database there may be some 404 error happening. Now we can use modern formats like avif, webp and jpeg together and browsers can choose the image based on support.
How to do a graceful degradation and show the fallback image if the avif or webp image is 404.
<script>
function onError() {
this.onerror = null;
this.parentNode.children[0].srcset = this.parentNode.children[1].srcset = this.src;
}
</script>
<picture>
<source srcset="picture-not-found1.avif" type="image/avif" />
<source srcset="picture-not-found1.webp" type="image/webp" />
<img src="fallback.jpeg" alt="my image" onerror="onError.call(this)" />
</picture>
We can do a one line onerror also if you don't want a common function for all images.
<picture>
<source srcset="picture-not-found2.avif" type="image/avif" />
<source srcset="picture-not-found2.webp" type="image/webp" />
<img src="fallback.jpeg" alt="my image" onerror="this.onerror = null;this.parentNode.children[0].srcset = this.parentNode.children[1].srcset = this.src;" />
</picture>
If you have only one source you can change the function as below
<picture>
<source srcset="picture-not-found2.webp" type="image/webp" />
<img src="fallback.jpeg" alt="" onerror="this.onerror = null;this.parentNode.children[0].srcset = this.src;" />
</picture>
Stackoverflow Answers
Thanks for reading
Cheers ✌🏻